Generate custom API Client for Shopware 6
Why?
With Composable Frontends, you can create your own JavaScript/Typescript based API Client from your local or online running Shopware 6 Instance. This brings you the advantage that all Custom Endpoints are also included in the API Client if they have a proper OpenAPI Schema definition. In this post, I describe how and what steps are needed to do so. There will also be another blog post about how to extend the paths and operations manually.
Requirements
- A running Shopware 6 Instance (local or online)
- A cloned Composable Frontends Repository
Process to generate Schema for store API Client
Steps to generate storeApiSchema.json and storeApiSchema.d.ts files
- From your Frontends Repository folder go into this sub-folder packages/api-gen
- Make sure your API schema JSON file is reachable
(e.g. http://127.0.0.1:8000/store-api/_info/openapi3.json) - Create a .env File with these values:
OPENAPI_JSON_URL="http://127.0.0.1:8000"
OPENAPI_ACCESS_KEY="YOUR_ACCESS_KEY" - Download the schema file with this command (executed in the api-gen folder):
pnpm cli loadSchema --apiType=store --filename=storeApiSchema.json
Now there should be a new file in packages/api-gen/storeApiSchema.json - Create the types from the downloaded schema file with that command:
pnpm cli generate --filename=storeApiSchema.json
Now there should be a new file in packages/api-gen/storeApiSchema.d.ts
(better rename to customStoreApiTypes-6.5.8.3.d.ts, including your Shopware Version number)
Now you can import your custom operations and paths in code like this:
import type {
operationPaths,
operations,
components,
} from "../path/to/your/file/storeApiSchema";
Process to generate Schema for admin API Client
Steps to generate adminApiSchema.json and adminApiSchema.d.ts files
- From your Frontends Repository folder go into this sub-folder packages/api-gen
- Make sure your API schema JSON file is reachable
(e.g. http://127.0.0.1:8000/api/_info/openapi3.json) - Create a .env File with these values:
OPENAPI_JSON_URL="http://127.0.0.1:8000"
SHOPWARE_ADMIN_USERNAME="admin"
SHOPWARE_ADMIN_PASSWORD="shopware" - Download the schema file with this command (executed in the api-gen folder):
pnpm cli loadSchema --apiType=admin --filename=adminApiSchema.json
Now there should be a new file in packages/api-gen/adminApiSchema.json - Create the types from the downloaded schema file with that command:
pnpm cli generate --filename=adminApiSchema.json
Now there should be a new file in packages/api-gen/adminApiSchema.d.ts
(better rename to customAdminApiTypes-6.5.8.3.d.ts, including your Shopware Version number)
Now you can import your custom operations and paths in code like this:
import type {
operationPaths,
operations,
components,
} from "../path/to/your/file/adminApiSchema";
You do not want to generate your own Schema Files?
Just use the ones that are provided via the API Client package.
For store API:
import type {
operationPaths,
operations,
components,
} from "@shopware/api-client/api-types";
For admin API:
import type {
operationPaths,
operations,
components,
} from "@shopware/api-client/admin-api-types";
Compare OpenAPI Schema’s
Steps to compare new and old apiSchema files to see the changes between versions for example.
- Make sure you have Golang installed and it is working in your terminal (export path)
- We will use oasdiff to compare the OpenAPI schema JSON files
- Install oasdiff with this command:
go install github.com/tufin/oasdiff@latest - Create two different apiSchema.json files for example one for Shopware 6.6. and one for Shopware 6.5 (see generate steps above)
- Now execute this diff command where the two different JSON files are located:
oasdiff diff apiSchema-before.json apiSchema.json -f html > result.html - Now you can open the result.html in your browser and look through the changes.
Example how the result.html Page will look like
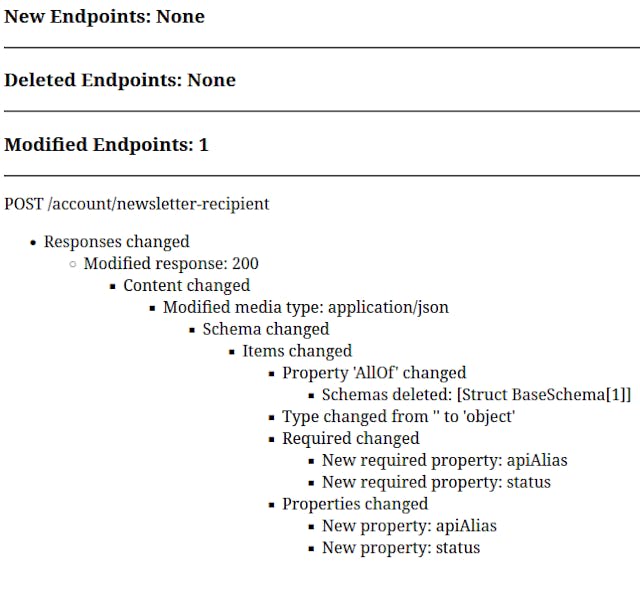
Known Issues
- Do not use this CLI command for generating the schema JSON file:
bin/console framework:schema -s openapi3 --store-api apiSchema.json
Because the component's schema definitions are missing.